Get Status
getStatus
Fetches the status of an ongoing swap based on the provided swapStatusRequest
argument.
getStatus(
swapStatusRequest: SwapStatusRequest,
options?: RequestOptions
): Promise<SwapStatusResponse>
The SwapStatusRequest
object includes the following arguments:
Param | Description | Data type |
---|---|---|
id (required) | One of the following:
| string |
The response will include the following:
interface SwapFee {
type: 'LIQUIDITY' | 'NETWORK' | 'INGRESS' | 'EGRESS' | 'BROKER' | 'BOOST';
chain: Chain;
asset: Asset;
amount: string;
}
interface CommonStatusFields {
srcChain: Chain;
destChain: Chain;
srcAsset: Asset;
destAsset: Asset;
destAddress: string | undefined;
depositAddress: string | undefined;
srcChainRequiredBlockConfirmations: number | undefined;
depositChannelBrokerCommissionBps: number | undefined;
depositChannelAffiliateBrokers: {
account: string;
commissionBps: number
}[] | undefined;
estimatedDepositChannelExpiryTime: number | undefined;
expectedDepositAmount: string | undefined;
isDepositChannelExpired: boolean;
ccmDepositReceivedBlockIndex: string | undefined;
ccmParams: {
gasBudget: string;
message: `0x${string}`;
} | undefined;
feesPaid: SwapFee[];
depositChannelMaxBoostFeeBps: number;
effectiveBoostFeeBps: number | undefined;
depositBoostedAt: number | undefined;
depositBoostedBlockIndex: string | undefined;
boostSkippedAt: number | undefined;
boostSkippedBlockIndex: string | undefined;
fillOrKillParams: {
retryDurationBlocks: number,
refundAddress: string,
minPrice: string
} | undefined
}
export type SwapStatusResponse = CommonStatusFields &
(
| {
// we are waiting for the user to send funds
state: 'AWAITING_DEPOSIT';
depositAmount: string | undefined;
depositTransactionRef: string | undefined;
depositTransactionConfirmations: number | undefined;
}
| {
// funds have been received and the swap is being performed
state: 'DEPOSIT_RECEIVED';
swapId: string;
depositAmount: string;
depositTransactionRef: string | undefined;
depositReceivedAt: number;
depositReceivedBlockIndex: string;
}
| {
// funds have been swapped through the AMM and awaiting scheduling
state: 'SWAP_EXECUTED';
swapId: string;
depositAmount: string;
depositTransactionRef: string | undefined;
depositReceivedAt: number;
depositReceivedBlockIndex: string;
intermediateAmount: string | undefined;
swapScheduledAt: number | undefined;
swapScheduledBlockIndex: string | undefined;
swapExecutedAt: number;
swapExecutedBlockIndex: string;
}
| {
// funds have been scheduled to be sent to the destination address (or refunded to the refund address)
state: 'EGRESS_SCHEDULED';
egressType: 'SWAP' | 'REFUND';
swapId: string;
depositAmount: string;
depositTransactionRef: string | undefined;
depositReceivedAt: number;
depositReceivedBlockIndex: string;
intermediateAmount: string | undefined;
swapScheduledAt: number | undefined;
swapScheduledBlockIndex: string | undefined;
swapExecutedAt: number | undefined;
swapExecutedBlockIndex: string | undefined;
egressAmount: string;
egressScheduledAt: number;
egressScheduledBlockIndex: string;
}
| {
state:
// a validator has been requested to send the funds
| "BROADCAST_REQUESTED"
// the transaction has been included in a block on the respective chain
| "BROADCASTED";
egressType: 'SWAP' | 'REFUND';
swapId: string;
depositAmount: string;
depositTransactionRef: string | undefined;
depositReceivedAt: number;
depositReceivedBlockIndex: string;
intermediateAmount: string | undefined;
swapScheduledAt: number | undefined;
swapScheduledBlockIndex: string | undefined;
swapExecutedAt: number;
swapExecutedBlockIndex: string;
egressAmount: string;
egressScheduledAt: number;
egressScheduledBlockIndex: string;
broadcastRequestedAt: number;
broadcastRequestedBlockIndex: string;
broadcastTransactionRef: string;
}
| {
// the transaction could not be successfully completed
state: 'BROADCAST_ABORTED';
egressType: 'SWAP' | 'REFUND';
swapId: string;
depositAmount: string;
depositTransactionRef: string | undefined;
depositReceivedAt: number;
depositReceivedBlockIndex: string;
intermediateAmount: string | undefined;
swapScheduledAt: number | undefined;
swapScheduledBlockIndex: string | undefined;
swapExecutedAt: number;
swapExecutedBlockIndex: string;
egressAmount: string;
egressScheduledAt: number;
egressScheduledBlockIndex: string;
broadcastRequestedAt: number;
broadcastRequestedBlockIndex: string;
broadcastAbortedAt: number;
broadcastAbortedBlockIndex: string;
}
| {
// the transaction has been confirmed beyond our safety margin
state: 'COMPLETE';
egressType: 'SWAP' | 'REFUND';
swapId: string;
depositAmount: string;
depositTransactionRef: string | undefined;
depositReceivedAt: number;
depositReceivedBlockIndex: string;
intermediateAmount: string | undefined;
swapScheduledAt: number | undefined;
swapScheduledBlockIndex: string | undefined;
swapExecutedAt: number;
swapExecutedBlockIndex: string;
egressAmount: string;
egressScheduledAt: number;
egressScheduledBlockIndex: string;
broadcastRequestedAt: number;
broadcastRequestedBlockIndex: string;
broadcastSucceededAt: number;
broadcastSucceededBlockIndex: string;
broadcastTransactionRef: string;
}
| {
// the swap failed due to the deposit being too low
state: 'FAILED';
failure: 'INGRESS_IGNORED';
error: { name: string; message: string };
depositAmount: string;
depositTransactionRef: string | undefined;
failedAt: number;
failedBlockIndex: string;
}
| {
// the swap failed due to the swap output being too low
state: 'FAILED';
failure: 'EGRESS_IGNORED';
error: { name: string; message: string };
swapId: string;
depositAmount: string;
depositTransactionRef: string | undefined;
depositReceivedAt: number;
depositReceivedBlockIndex: string;
intermediateAmount: string | undefined;
swapExecutedAt: number;
swapExecutedBlockIndex: string;
ignoredEgressAmount: string;
egressIgnoredAt: number;
egressIgnoredBlockIndex: string;
}
| {
// the swap failed doe the to refund amount being too low
state: 'FAILED';
failure: 'REFUND_EGRESS_IGNORED';
error: { name: string; message: string };
swapId: string;
depositAmount: string;
depositReceivedAt: number;
depositReceivedBlockIndex: string;
ignoredEgressAmount: string;
egressIgnoredAt: number;
egressIgnoredBlockIndex: string;
}
);
depositTransactionRef
and broadcastTransactionRef
The depositTransactionRef
property references the transaction that triggered a swap,
if the Chainflip protocol was able to identify a single transaction. The broadcastTransactionRef
property references the transaction that sent the swapped assets to the user.
These fields have a different format depending on the chain of the transaction:
- For Polkadot, the transaction reference is in the format
${blockNumber}-${extrinsicIndex}
. For example,200-3
refers to the fourth extrinsic in block 200. - For EVM-based chains, the transaction reference is the transaction hash.
- For Bitcoin, the transaction reference is the transaction ID.
Example
Here is an example using Request Deposit Address:
const swapStatusRequest = {
id: "1234567890", // depositChannelId or transactionHash
};
console.log(await swapSDK.getStatus(swapStatusRequest));
Sample Response
{
"state": "COMPLETE",
"type": "SWAP",
"srcChain": "Bitcoin",
"srcAsset": "BTC",
"destChain": "Ethereum",
"destAsset": "ETH",
"destAddress": "0x7364e83c8d74a1296101515ac91297e5344fdf71",
"depositChannelCreatedAt": 1716897288103,
"depositChannelBrokerCommissionBps": 0,
"depositAddress": "bcrt1pvxr5tt29f7eka7u4lc9gujg7zvzpye09txsm3gvhm792m30vfvpq9xr0k6",
"expectedDepositAmount": "10000000", // 0.1 BTC
"swapId": "21",
"depositAmount": "1000000", // 0.1 BTC
"depositTransactionRef": "c641a78ca139a727c1c38b48f8b2abf4a7c6bc2a3331a20f533fd0a532b47a32",
"depositReceivedAt": 1716897318000,
"depositReceivedBlockIndex": "31704-10",
"intermediateAmount": "98067254", // 98.06 USDC
"swapExecutedAt": 1716897330000,
"swapExecutedBlockIndex": "31706-39",
"egressAmount": "99884842264797301", // 0.09988 ETH
"egressScheduledAt": 1716897330000,
"egressScheduledBlockIndex": "31706-40",
"feesPaid": [
{
"type": "BOOST",
"chain": "Bitcoin",
"asset": "BTC",
"amount": "500"
},
{
"type": "INGRESS",
"chain": "Bitcoin",
"asset": "BTC",
"amount": "78"
},
{
"type": "NETWORK",
"chain": "Ethereum",
"asset": "USDC",
"amount": "98067"
},
{
"type": "LIQUIDITY",
"chain": "Bitcoin",
"asset": "BTC",
"amount": "19"
},
{
"type": "LIQUIDITY",
"chain": "Ethereum",
"asset": "USDC",
"amount": "1961"
},
{
"type": "EGRESS",
"chain": "Ethereum",
"asset": "ETH",
"amount": "105000000490000"
}
],
"broadcastRequestedAt": 1716897330000,
"broadcastRequestedBlockIndex": "31706-42",
"broadcastSucceededAt": 1716897468000,
"broadcastSucceededBlockIndex": "31729-25",
"depositChannelExpiryBlock": "37571",
"estimatedDepositChannelExpiryTime": 1716972270000,
"isDepositChannelExpired": false,
"depositChannelOpenedThroughBackend": true,
"broadcastTransactionRef": "0x4c33e1e69609f4d9c918b5452282b9539718fe13c34aec02a38a181f746d35ec", // in case the destination chain is polkadot, the format of this tx ref is "{block_number}-{extrinsic_index}" (ex. "20054255-2")
"depositChannelMaxBoostFeeBps": 30,
"effectiveBoostFeeBps": 5
}
Lifecycle of a normal swap
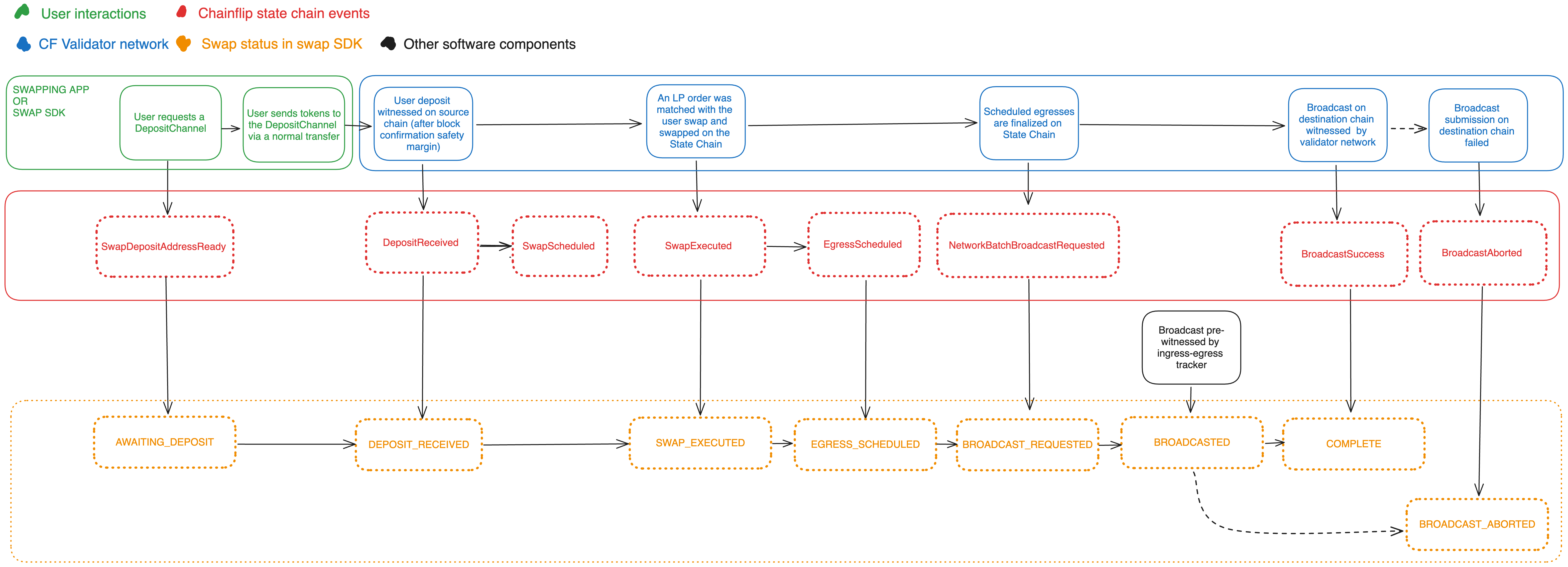
Lifecycle of a boosted swap
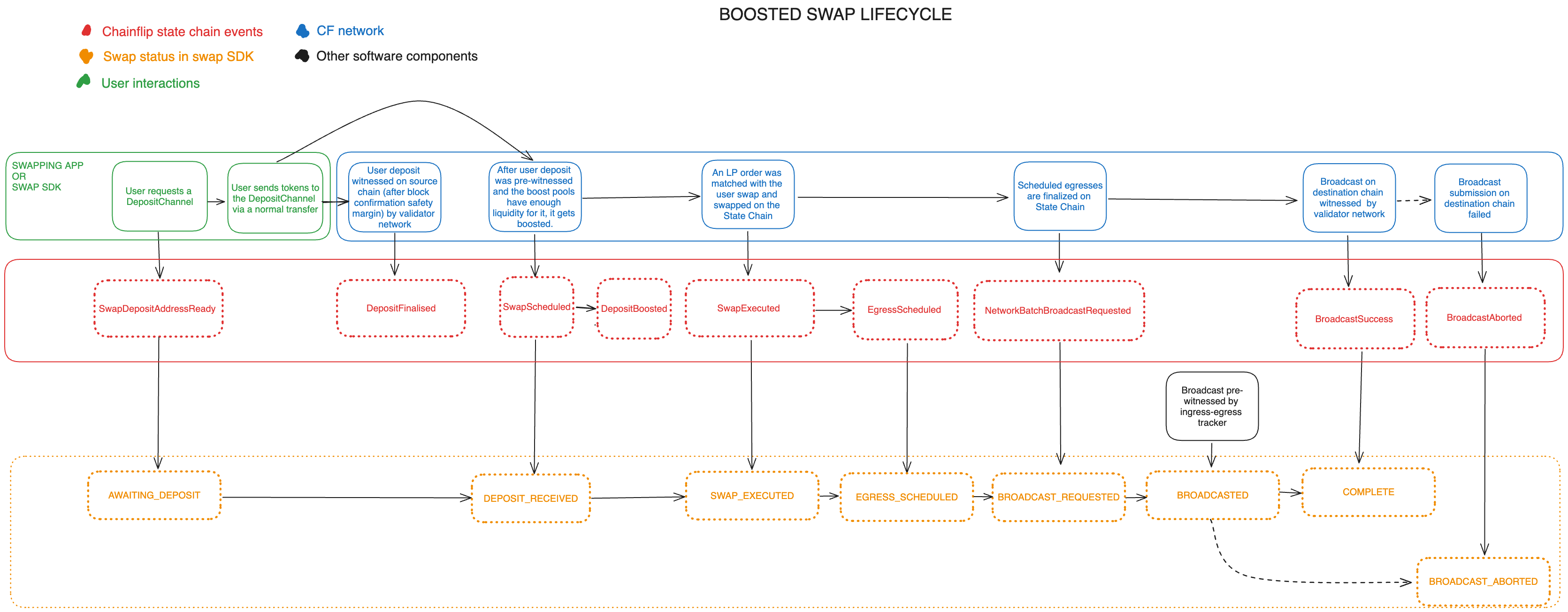