Get Status V2
getStatusV2
Fetches the status of an ongoing swap based on the provided swapStatusRequest
argument.
getStatusV2(
swapStatusRequest: SwapStatusRequest,
options?: RequestOptions
): Promise<SwapStatusResponseV2>
The SwapStatusRequest
object includes the following arguments:
Param | Description | Data type |
---|---|---|
id (required) | One of the following:
| string |
The SwapStatusRequestV2
response will include the following:
interface Boost {
maxBoostFeeBps: number;
effectiveBoostFeeBps: number | undefined;
boostedAt: number | undefined;
boostedBlockIndex: string | undefined;
skippedAt: number | undefined;
skippedBlockIndex: string | undefined;
}
interface DepositChannelFields {
id: string;
createdAt: number;
brokerCommissionBps: number;
depositAddress: string;
srcChainExpiryBlock: string;
estimatedExpiryTime: number;
expectedDepositAmount: string;
isExpired: boolean;
openedThroughBackend: boolean;
affiliateBrokers: AffiliateBroker[];
fillOrKillParams: FillOrKillParams | undefined;
dcaParams: DcaParams | undefined;
}
interface DepositFields {
amount: string;
txRef: string | undefined;
txConfirmations: number | undefined;
witnessedAt: number | undefined;
witnessedBlockIndex: string | undefined;
failure: Failure | undefined;
failedAt: number | undefined;
failedBlockIndex: string | undefined;
}
interface PaidFee {
type: 'NETWORK' | 'INGRESS' | 'EGRESS' | 'BROKER' | 'BOOST' | 'LIQUIDITY';
chain: Chain;
asset: Asset;
amount: string;
}
interface SwapFields {
originalInputAmount: string;
remainingInputAmount: string;
swappedInputAmount: string;
swappedOutputAmount: string;
regular?: {
inputAmount: string;
outputAmount: string;
scheduledAt: number;
scheduledBlockIndex: string;
executedAt?: number;
executedBlockIndex?: string;
retryCount: 0;
};
dca?: {
lastExecutedChunk: {
inputAmount: string;
outputAmount: string;
scheduledAt: number;
scheduledBlockIndex: string;
executedAt: number;
executedBlockIndex: string;
retryCount: number;
} | null;
currentChunk: {
inputAmount: string;
scheduledAt: number;
scheduledBlockIndex: string;
retryCount: number;
} | null;
executedChunks: number;
remainingChunks: number;
};
}
interface EgressFields {
amount: string;
scheduledAt: number;
scheduledBlockIndex: string;
txRef: string | undefined;
witnessedAt: number | undefined;
witnessedBlockIndex: string | undefined;
failure: Failure | undefined;
failedAt: number | undefined;
failedBlockIndex: string | undefined;
}
interface SwapStatusResponseCommonFields {
swapId: string;
srcChain: Chain;
srcAsset: Asset;
destChain: Chain;
destAsset: Asset;
destAddress: string;
depositChannel: DepositChannelFields | undefined;
ccmParams: CcmParams | undefined;
boost: Boost | undefined;
fees: PaidFee[];
estimatedDurationSeconds: number | null | undefined; // total estimated time until destination asset is received by the user
estimatedDurationsSeconds: {
deposit: number; // estimated time for a deposit to be witnessed
swap: number; // estimated time for a swap to be fully executed
egress: number; // estimated time until the output transaction is included in a block
};
srcChainRequiredBlockConfirmations: number | null;
}
interface Waiting extends SwapStatusResponseCommonFields {
depositChannel: DepositChannelFields;
}
interface Receiving extends SwapStatusResponseCommonFields {
deposit: DepositFields;
}
interface Swapping extends Receiving {
swap: SwapFields;
}
interface Sending extends Receiving {
swap: SwapFields;
swapEgress: EgressFields | undefined;
refundEgress: EgressFields | undefined;
}
export type SwapStatusResponseV2 =
| ({
state: 'WAITING';
} & Waiting)
| ({
state: 'RECEIVING';
} & Receiving)
| ({
state: 'SWAPPING';
} & Swapping)
| ({
state: 'SENDING';
} & Sending)
| ({
state: 'SENT';
} & Sending)
| ({
state: 'COMPLETED';
} & Sending)
| ({
state: 'FAILED';
Example
Here is an example using Request Deposit Address:
const swapStatusRequest = {
id: "1234567890", // depositChannelId or transactionHash
};
console.log(await swapSDK.getStatusV2(swapStatusRequest));
Sample Responses
DCA Swap
{
"state": "COMPLETED",
"srcAsset": "ETH",
"srcChain": "Ethereum",
"destAsset": "DOT",
"destChain": "Polkadot",
"destAddress": "1yMmfLti1k3huRQM2c47WugwonQMqTvQ2GUFxnU7Pcs7xPo",
"estimatedDurationSeconds": 102,
"estimatedDurationsSeconds": {
"deposit": 84,
"swap": 12,
"egress": 6
},
"srcChainRequiredBlockConfirmations": 2,
"deposit": {
"amount": "9999949999999650000",
"txRef": "0x1e6e4a865a18aa31306e088966e14193f95f409e40830b829963c365207596ce",
"witnessedAt": 552000,
"witnessedBlockIndex": "92-400",
},
"depositChannel": {
"brokerCommissionBps": 0,
"createdAt": 516000,
"dcaParams": {
"chunkIntervalBlocks": 3,
"numberOfChunks": 10,
},
"depositAddress": "0x6aa69332b63bb5b1d7ca5355387edd5624e181f2",
"estimatedExpiryTime": 1699527060000,
"id": "86-Ethereum-85",
"isExpired": false,
"openedThroughBackend": false,
"srcChainExpiryBlock": "265",
},
"fees": [
{
"amount": "9112484",
"asset": "USDC",
"chain": "Ethereum",
"type": "NETWORK",
},
{
"amount": "9999899999999300",
"asset": "ETH",
"chain": "Ethereum",
"type": "LIQUIDITY",
},
{
"amount": "9012338",
"asset": "USDC",
"chain": "Ethereum",
"type": "LIQUIDITY",
},
{
"amount": "91033720",
"asset": "ETH",
"chain": "Ethereum",
"type": "BROKER",
},
{
"amount": "50000000350000",
"asset": "ETH",
"chain": "Ethereum",
"type": "INGRESS",
},
{
"amount": "197450000",
"asset": "DOT",
"chain": "Polkadot",
"type": "EGRESS",
},
],
"swap": {
"originalInputAmount": "9999899999999300000",
"remainingInputAmount": "0",
"swappedInputAmount": "9999899999999300000",
"swappedOutputAmount": "8385809332068",
"dca": {
"currentChunk": null,
"executedChunks": 2,
"lastExecutedChunk": {
"executedAt": 564000,
"executedBlockIndex": "94-594",
"inputAmount": "4999949999999650000",
"outputAmount": "4192904666034",
"retryCount": 0,
"scheduledAt": 552000,
"scheduledBlockIndex": "92-399",
},
"remainingChunks": 8,
},
},
"swapEgress": {
"amount": "4192707216034",
"scheduledAt": 564000,
"scheduledBlockIndex": "94-595",
"txRef": "104-2",
"witnessedAt": 624000,
"witnessedBlockIndex": "104-7",
},
}
Regular Swap
{
"state": "COMPLETED",
"srcChain": "Ethereum",
"srcAsset": "ETH",
"destChain": "Polkadot",
"destAsset": "DOT",
"destAddress": "1yMmfLti1k3huRQM2c47WugwonQMqTvQ2GUFxnU7Pcs7xPo",
"estimatedDurationSeconds": 102,
"estimatedDurationsSeconds": {
"deposit": 84,
"swap": 12,
"egress": 6
},
"srcChainRequiredBlockConfirmations": 2,
"deposit": {
"amount": "5000000000000000000",
"txRef": "0x1e6e4a865a18aa31306e088966e14193f95f409e40830b829963c365207596ce",
"witnessedAt": 552000,
"witnessedBlockIndex": "92-400",
},
"depositChannel": {
"id": "86-Ethereum-85",
"createdAt": 516000,
"depositAddress": "0x6aa69332b63bb5b1d7ca5355387edd5624e181f2",
"isExpired": false,
"brokerCommissionBps": 0,
"estimatedExpiryTime": 1699527060000,
"openedThroughBackend": false,
"srcChainExpiryBlock": "265",
},
"fees": [
{
"amount": "4556242",
"asset": "USDC",
"chain": "Ethereum",
"type": "NETWORK",
},
{
"amount": "4999949999999650",
"asset": "ETH",
"chain": "Ethereum",
"type": "LIQUIDITY",
},
{
"amount": "4506169",
"asset": "USDC",
"chain": "Ethereum",
"type": "LIQUIDITY",
},
{
"amount": "45516860",
"asset": "ETH",
"chain": "Ethereum",
"type": "BROKER",
},
{
"amount": "197450000",
"asset": "DOT",
"chain": "Polkadot",
"type": "EGRESS",
},
],
"swap": {
"originalInputAmount": "4999949999999650000",
"remainingInputAmount": "0",
"swappedInputAmount": "4999949999999650000",
"swappedOutputAmount": "4192904666034",
"regular": {
"executedAt": 564000,
"executedBlockIndex": "94-594",
"inputAmount": "4999949999999650000",
"outputAmount": "4192904666034",
"retryCount": 0,
"scheduledAt": 552000,
"scheduledBlockIndex": "92-399",
},
},
"swapEgress": {
"amount": "4192707216034",
"scheduledAt": 564000,
"scheduledBlockIndex": "94-595",
"txRef": "104-2",
"witnessedAt": 624000,
"witnessedBlockIndex": "104-7",
},
}
Boost Swap
{
"state": "COMPLETED",
"srcChain": "Ethereum",
"srcAsset": "ETH",
"destChain": "Polkadot",
"destAsset": "DOT",
"estimatedDurationSeconds": 102,
"estimatedDurationsSeconds": {
"deposit": 84,
"swap": 12,
"egress": 6
},
"srcChainRequiredBlockConfirmations": 2,
"destAddress": "1yMmfLti1k3huRQM2c47WugwonQMqTvQ2GUFxnU7Pcs7xPo",
"depositChannel": {
"brokerCommissionBps": 0,
"createdAt": 516000,
"depositAddress": "0x6aa69332b63bb5b1d7ca5355387edd5624e181f2",
"estimatedExpiryTime": 1699527060000,
"id": "86-Ethereum-85",
"isExpired": false,
"openedThroughBackend": false,
"srcChainExpiryBlock": "265",
},
"deposit": {
"amount": "5000000000000000000",
"txRef": "0x1e6e4a865a18aa31306e088966e14193f95f409e40830b829963c365207596ce",
"witnessedAt": 552000,
"witnessedBlockIndex": "92-400",
},
"boost": {
"boostedAt": 552000,
"boostedBlockIndex": "92-400",
"effectiveBoostFeeBps": 5,
"maxBoostFeeBps": 30,
},
"fees": [
{
"amount": "4556242",
"asset": "USDC",
"chain": "Ethereum",
"type": "NETWORK",
},
{
"amount": "4999949999999650",
"asset": "ETH",
"chain": "Ethereum",
"type": "LIQUIDITY",
},
{
"amount": "4506169",
"asset": "USDC",
"chain": "Ethereum",
"type": "LIQUIDITY",
},
{
"amount": "45516860",
"asset": "ETH",
"chain": "Ethereum",
"type": "BROKER",
},
{
"amount": "2500000000000000",
"asset": "ETH",
"chain": "Ethereum",
"type": "BOOST",
},
{
"amount": "50000000350000",
"asset": "ETH",
"chain": "Ethereum",
"type": "INGRESS",
},
{
"amount": "50000000350000",
"asset": "ETH",
"chain": "Ethereum",
"type": "INGRESS",
},
{
"amount": "197450000",
"asset": "DOT",
"chain": "Polkadot",
"type": "EGRESS",
},
],
"swap": {
"originalInputAmount": "4997399999999300000",
"regular": {
"executedAt": 564000,
"executedBlockIndex": "94-594",
"inputAmount": "4999949999999650000",
"outputAmount": "4192904666034",
"retryCount": 0,
"scheduledAt": 552000,
"scheduledBlockIndex": "92-399",
},
"remainingInputAmount": "0",
"swappedInputAmount": "4999949999999650000",
"swappedOutputAmount": "4192904666034",
},
"swapEgress": {
"amount": "4192707216034",
"scheduledAt": 564000,
"scheduledBlockIndex": "94-595",
"txRef": "104-2",
"witnessedAt": 624000,
"witnessedBlockIndex": "104-7",
},
}
Lifecycle of a swap
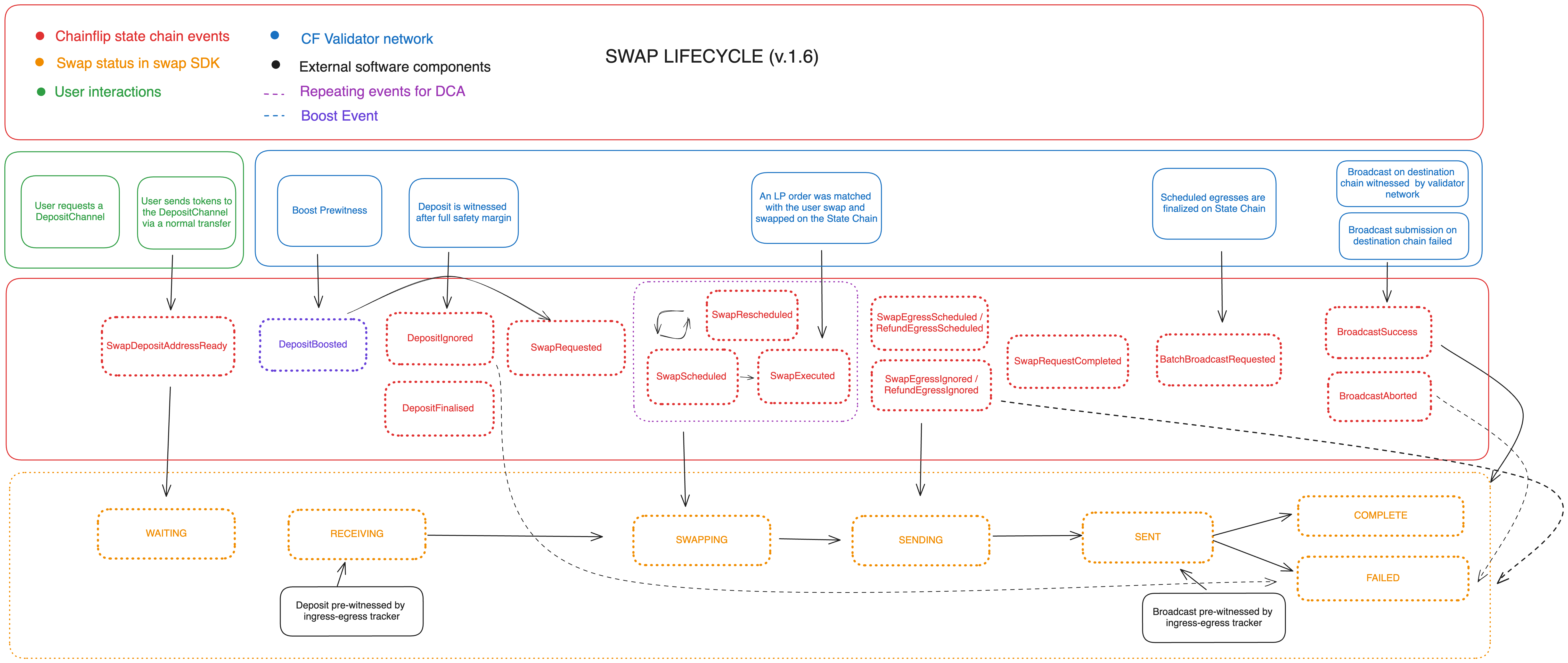