Get Status
getStatusV2
Fetches the status of an ongoing swap based on the provided swapStatusRequest
argument.
function getStatusV2(swapStatusRequest: SwapStatusRequest, options?: RequestOptions): Promise<SwapStatusResponseV2>
type SwapStatusRequest = {
id: string;
};
type RequestOptions = {
signal?: AbortSignal;
}
The SwapStatusRequest
object includes the following arguments:
Param | Description | Data type |
---|---|---|
id (required) | One of the following:
| string |
Result type
interface Boost {
maxBoostFeeBps: number;
effectiveBoostFeeBps: number | undefined;
boostedAt: number | undefined;
boostedBlockIndex: string | undefined;
skippedAt: number | undefined;
skippedBlockIndex: string | undefined;
}
interface DepositChannelFields {
id: string;
createdAt: number;
brokerCommissionBps: number;
depositAddress: string;
srcChainExpiryBlock: string;
estimatedExpiryTime: number;
expectedDepositAmount: string;
isExpired: boolean;
openedThroughBackend: boolean;
affiliateBrokers: AffiliateBroker[];
fillOrKillParams: FillOrKillParams | undefined;
dcaParams: DcaParams | undefined;
}
interface DepositFields {
amount: string;
txRef: string | undefined;
txConfirmations: number | undefined;
witnessedAt: number | undefined;
witnessedBlockIndex: string | undefined;
failure: Failure | undefined;
failedAt: number | undefined;
failedBlockIndex: string | undefined;
}
interface PaidFee {
type: 'NETWORK' | 'INGRESS' | 'EGRESS' | 'BROKER' | 'BOOST' | 'LIQUIDITY';
chain: Chain;
asset: Asset;
amount: string;
}
interface SwapFields {
originalInputAmount: string;
remainingInputAmount: string;
swappedInputAmount: string;
swappedOutputAmount: string;
regular?: {
inputAmount: string;
outputAmount: string;
scheduledAt: number;
scheduledBlockIndex: string;
executedAt?: number;
executedBlockIndex?: string;
retryCount: 0;
};
dca?: {
lastExecutedChunk: {
inputAmount: string;
outputAmount: string;
scheduledAt: number;
scheduledBlockIndex: string;
executedAt: number;
executedBlockIndex: string;
retryCount: number;
} | null;
currentChunk: {
inputAmount: string;
scheduledAt: number;
scheduledBlockIndex: string;
retryCount: number;
} | null;
executedChunks: number;
remainingChunks: number;
};
}
interface EgressFields {
amount: string;
scheduledAt: number;
scheduledBlockIndex: string;
txRef: string | undefined;
witnessedAt: number | undefined;
witnessedBlockIndex: string | undefined;
failure: Failure | undefined;
failedAt: number | undefined;
failedBlockIndex: string | undefined;
}
interface SwapStatusResponseCommonFields {
swapId: string;
srcChain: Chain;
srcAsset: Asset;
destChain: Chain;
destAsset: Asset;
destAddress: string;
depositChannel: DepositChannelFields | undefined;
ccmParams: CcmParams | undefined;
boost: Boost | undefined;
fees: PaidFee[];
estimatedDurationSeconds: number | null | undefined; // total estimated time until destination asset is received by the user
estimatedDurationsSeconds: {
deposit: number; // estimated time for a deposit to be witnessed
swap: number; // estimated time for a swap to be fully executed
egress: number; // estimated time until the output transaction is included in a block
};
srcChainRequiredBlockConfirmations: number | null;
}
interface Waiting extends SwapStatusResponseCommonFields {
depositChannel: DepositChannelFields;
}
interface Receiving extends SwapStatusResponseCommonFields {
deposit: DepositFields;
}
interface Swapping extends Receiving {
swap: SwapFields;
}
interface Sending extends Receiving {
swap: SwapFields;
swapEgress: EgressFields | undefined;
refundEgress: EgressFields | undefined;
}
export type SwapStatusResponseV2 =
| ({
state: 'WAITING';
} & Waiting)
| ({
state: 'RECEIVING';
} & Receiving)
| ({
state: 'SWAPPING';
} & Swapping)
| ({
state: 'SENDING';
} & Sending)
| ({
state: 'SENT';
} & Sending)
| ({
state: 'COMPLETED';
} & Sending)
| ({
state: 'FAILED';
} & Sending);
Example
const swapStatusRequest = {
id: '6726421-Bitcoin-34009', // depositChannelId / swapId / transactionId
};
console.log(await swapSDK.getStatusV2(swapStatusRequest));
// console output:
{
state: 'COMPLETED',
swapId: '332925',
srcAsset: 'BTC',
srcChain: 'Bitcoin',
destAsset: 'USDC',
destChain: 'Ethereum',
destAddress: '0xbbfefb09727136a6c3ddd28bd4abdf259b9351c1',
srcChainRequiredBlockConfirmations: 3,
estimatedDurationsSeconds: { deposit: 1806, swap: 12, egress: 102 },
estimatedDurationSeconds: 1920,
brokers: [
{ account: 'cFJZVRaybb2PBwxTiAiRLiQfHY4KPB3RpJK22Q7Fhqk979aCH', commissionBps: 5 },
{ account: 'cFK6mYjpajcwPDZ7JUsac8XUoVSJnhjL43ZMZW7YoN8HE4dD8', commissionBps: 60 },
],
fees: [
{ type: 'NETWORK', chain: 'Ethereum', asset: 'USDC', amount: '52965555' },
{ type: 'LIQUIDITY', chain: 'Bitcoin', asset: 'BTC', amount: '27972' },
{ type: 'BROKER', chain: 'Ethereum', asset: 'USDC', amount: '343931842' },
{ type: 'BOOST', chain: 'Bitcoin', asset: 'BTC', amount: '28000' },
{ type: 'INGRESS', chain: 'Bitcoin', asset: 'BTC', amount: '1251' },
{ type: 'EGRESS', chain: 'Ethereum', asset: 'USDC', amount: '0' },
],
depositChannel: {
id: '6726421-Bitcoin-34009',
createdAt: 1740415452001,
brokerCommissionBps: 5,
depositAddress: 'bc1ppaxkhh5c65zypqtjl7s5j4lqqaund3lzaz27cwlhw7nvg0s84cwqv4q6pw',
srcChainExpiryBlock: '885280',
estimatedExpiryTime: 1740502800000,
isExpired: false,
openedThroughBackend: false,
affiliateBrokers: [
{ account: 'cFK6mYjpajcwPDZ7JUsac8XUoVSJnhjL43ZMZW7YoN8HE4dD8', commissionBps: 60 },
],
fillOrKillParams: {
refundAddress: 'bc1qstlcaqcds8xea7dtktdn47hng7c3lq3fa7vrtm',
minPrice: '92307.460172',
retryDurationBlocks: 150,
},
dcaParams: { numberOfChunks: 18, chunkIntervalBlocks: 2 },
},
deposit: {
amount: '55970749',
txRef: 'ba030cf99b7b01d9455c3ab87a5d87a301b67e91640fc9c76014c6e5b97986d7',
witnessedAt: 1740415836000,
witnessedBlockIndex: '6726483-536',
},
swap: {
originalInputAmount: '55970749',
remainingInputAmount: '0',
swappedInputAmount: '55970749',
swappedIntermediateAmount: '0',
swappedOutputAmount: '52568658562',
dca: {
lastExecutedChunk: {
inputAmount: '3109487',
outputAmount: '2916364871',
scheduledAt: 1740416046000,
scheduledBlockIndex: '6726517-2189',
executedAt: 1740416058000,
executedBlockIndex: '6726519-1073',
retryCount: 0,
},
currentChunk: null,
executedChunks: 18,
remainingChunks: 0,
},
},
swapEgress: {
amount: '52568658562',
scheduledAt: 1740416058000,
scheduledBlockIndex: '6726519-1074',
witnessedAt: 1740416262000,
witnessedBlockIndex: '6726552-640',
txRef: '0xc8646625910babc04b1612526c27fbac68aacf6cc489affbae69d1d430f5fcb1',
},
fillOrKillParams: {
refundAddress: 'bc1qstlcaqcds8xea7dtktdn47hng7c3lq3fa7vrtm',
minPrice: '92307.460172',
retryDurationBlocks: 150,
},
dcaParams: { numberOfChunks: 18, chunkIntervalBlocks: 2 },
boost: {
effectiveBoostFeeBps: 5,
maxBoostFeeBps: 0,
boostedAt: 1740415836000,
boostedBlockIndex: '6726483-536',
},
lastStatechainUpdateAt: 1740497470820,
}
Lifecycle of a swap
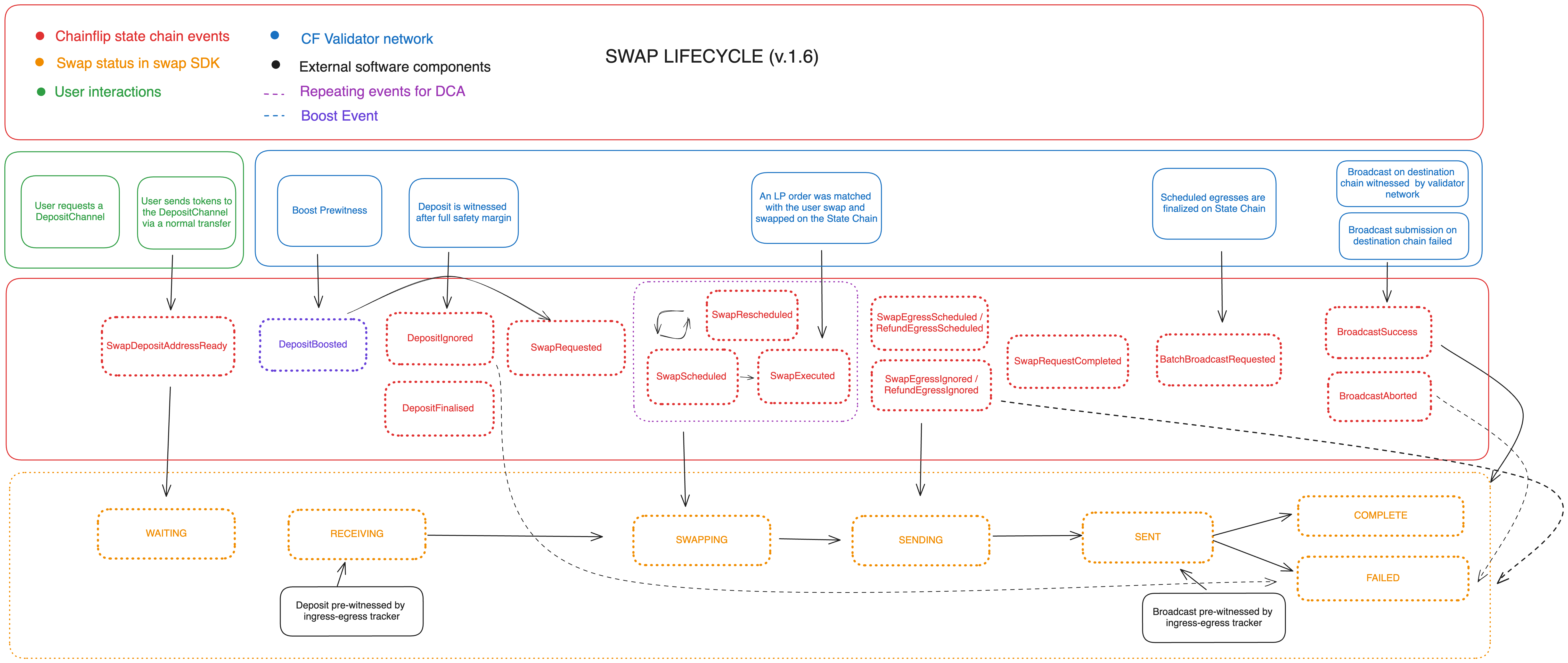